NPD I/O - NPD access library
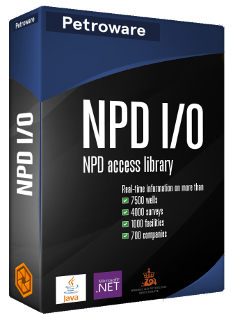
The Norwegian Petroleum Directorate (NPD) maintains a comprehensive database of the activities in the North Sea. This is public real-time information, updated on a daily basis.
NPD offers an extensive online interface to this information through their NPD fact pages, but in order to get hold of the actual data for performing statistics, advanced computations, visualizations or create custom applications, a library like NPD I/O is required. The NPD database contains information on more than 7500 wells, 4000 surveys, 120 fields, 1000 facilities, 700 companies, 1200 licenses and more.
NPD I/O is available for Java (NpdIo.jar).
The library is lightweight (< 100kB) and self-contained; It has no external dependencies.
NPD I/O access data directly through HTTP - no login or password is required.
API Documentation
![]() |
NPD I/O for Java: Javadoc |
![]() |
NPD I/O for .Net: Doxygen (on request) |
Coding example
Below are a few examples on how to access some of the main data types from the NPD database.
import no.petroware.npdio.well.*;
import no.petroware.npdio.field.*;
:
//
// Read all NPD development wellbores
//
List<NpdWellbore> wellbores = NpdDevelopmentWellboreReader.readAll();
// Loop over the wellbores and write to console
for (NpdWellbore wellbore : wellbores)
System.out.println(wellbore);
:
//
// Read all NPD fields
//
List fields = NpdFieldReader.readAll();
// Loop over the fields and load production data for each and write to console
for (NpdField field : fields) {
System.out.println(field.getName());
// Read production data
ProductionReader.readAll(field);
// Write oil production to console
for (Production.Entry productionEntry : field.getProduction().getEntries()) {
int year = productionEntry.getYear();
int month = productionEntry.getMonth();
double oilProduction = productionEntry.getOil();
System.out.println("Oil production: " + year + "/" + month + ": " + oilProduction);
}
}
:
Note that in an actual client implementation the reading process would better be done asynchronous in threads. The NPD I/O library is all thread-safe.
NPD I/O includes Java implementations for the NPD data types
wellbore,
license,
field,
company,
survey,
facility,
discovery,
business arrangement area,
pipeline and
stratigraphy.